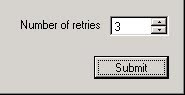
Specifying the control's Value property at design-time sets the starting value at runtime. If a user wants to set a different value, he must do so each time he starts the app. To make things more user-friendly it would be better to save the control's value between executions.
To do so, start by selecting the desired control in the designer. In the Properties window, expand the ApplicationSettings entry and select the ellipsis (...) for the PropertyBinding sub-entry.
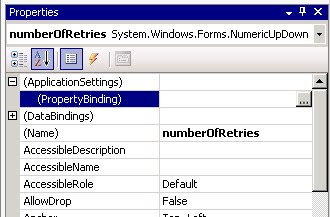
In the Application Settings dialog that appears, select the dropdown next to the 'Value' entry, as this is the property we wish to persist.
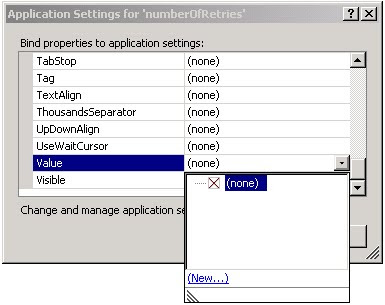
Select the '(New...)' link from the popup, which brings up the New Application Setting dialog.
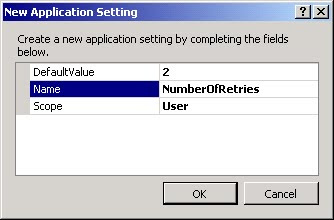
Specify the default value and name of the config setting (and modify the Scope if necessary) and press OK. This will update the Application Settings dialog to show the newly added entry.
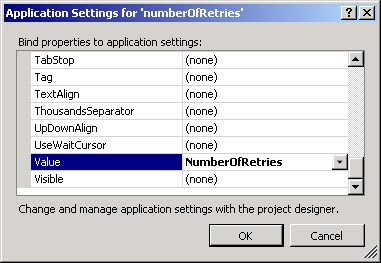
Press OK to close the dialog. With the property binding set, the application will automatically load the value at startup. The only thing left is to save the modified value. In the form's FormClosing event handler, add the following line:
Properties.Settings.Default.Save();
At this point the change is ready to test. Start the application and modify the control's value. Without doing anything else, close the application. Now restart the app. Note the control contains the modified value.
It would seem the desired functionality is complete but there is one more item that needs to address. If you look for the saved settings file, you will find it under
C:\Documents and Settings\<username>\Local Settings\Application Data\<Company>\<AppName>\<version>
If the version number for the application is changed, the previously saved setting won't be loaded. The application needs to know to upgrade the saved settings the first time it runs.
Start by adding a new application setting called UpgradeSettings. This will be used to make sure we only upgrade the settings once. Otherwise, any newly saved settings will be replaced by the previous version's settings every time the app starts.
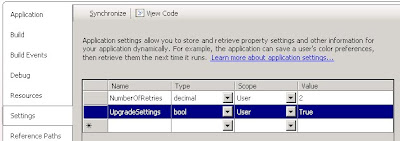
The final step is to add the upgrade logic to Program.cs. In the Main method, add the following code immediately before the call to Application.Run.
if (Convert.ToBoolean(Properties.Settings.Default["UpgradeSettings"]))
{
Properties.Settings.Default.Upgrade();
Properties.Settings.Default["UpgradeSettings"] = false;
}
No comments:
Post a Comment
Note: Only a member of this blog may post a comment.